JavaScript Recursive Function
Recursion is a process (function) that calls itself. One of many ways to approach a problem.
Let's do not be afraid of recursion, it can help us code with an elegant solution, keep code D.R.Y. and become a better developer. First of all, we already using recursion every day. In JSON.parse/JSON.stringify, DOM methods (getElementById…) under the hood a lot of recursive methods. So it is a very common practice in a developer’s life.
Recursive Factorial function
We are going to implement the most common example — factorial. A factorial is a function that multiplies a number by every number below it.
4! = 4 × 3 × 2 × 1 = 24
Let’s write a program to find factorial using recursion in JavaScript.
function factorial(n){
if(n === 0){ // base case
return 1
}
return n * factorial(n - 1) //invoke fn with different inputs
}
factorial(4) // 24
How does it work?
When we call a function factorial(n)
a compiler pushes it to the top of the call stack, every new call goes to the top. When the function ends or returns something compiler removes it from the top. Actually, we invoke the same function with different inputs until the base case. The base case is a stopping point when the function stops invoking.
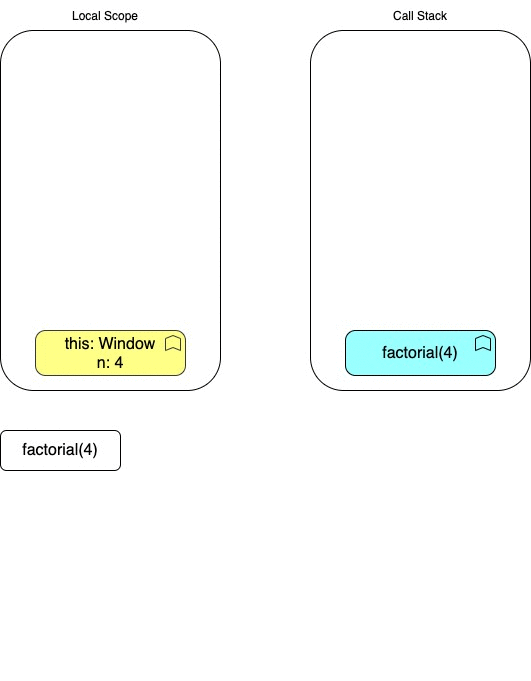
Every factorial function call waiting for a result from the previous call (on the top of the call stack) till we hit n === 0.
Recursion Flow
- Invoking function with different inputs
- Having base case, where is an exit from a function (return)
In recursion always have to be:
- Base Case
- Return correct value (recursive function should receive new input every call)
Recursion patterns
- Helper Method Recursion
- Function recursive itself — pure recursion
Helper Method Recursion is a pattern where defined outer (not recursive) function which calls an inner recursive function.
function evenNumbers (arr){
const res = [];
function helperFn(input){
if(input.length === 0) {
return
}
if(input[0] % 2 === 0){
res.push(input[0])
}
helperFn(input.slice(1))}
helperFn(arr)
return res
}
evenNumbers([2,3,5,6,79,4,2,4]) //[2, 6, 4, 2, 4]
Pure Recursion the function is totally self-contained in its recursive.
function evenNumbersPureRecursion(arr){
const res = []
if(arr.length === 0) return res
if(arr[0] % 2 === 0){
res.push(arr[0])
}
return [...res, ...evenNumbersPureRecursion(arr.splice(1))]
}
evenNumbersPureRecursion([2,3,5,6,79,4,2]) // [2, 6, 4, 2]